Learn how to resolve the Laravel unable to JSON encode payload error code 5. Our Laravel support services team is here to help you with your questions and concerns.
Laravel Unable to JSON Encode Payload Error Code 5
The error message laravel unable to json encode payload error code 5 is a frequent issue in Laravel, usually occurring when trying to queue a job or dispatch an event containing data that cannot be correctly serialized into JSON.
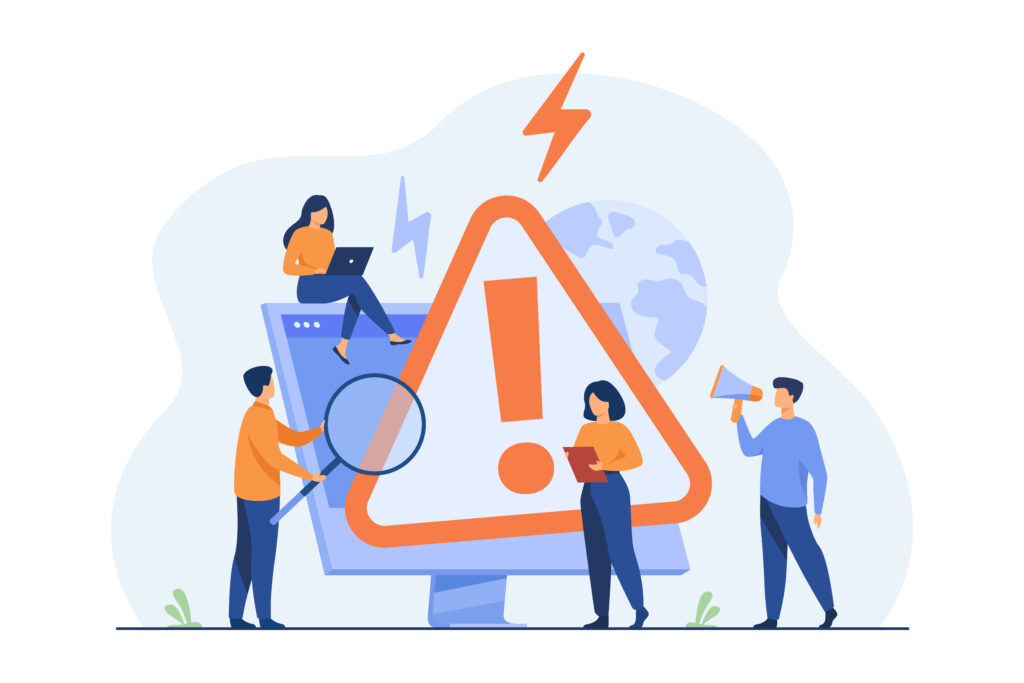
Error Syntax
The complete error message generally appears as:
Illuminate\Queue\InvalidPayloadException: Unable to JSON encode payload. Error code: 5
Impacts of the Error
Response Generation Failure
- Prevents the creation of JSON responses.
- Disrupts API endpoint functionality.
- Blocks data exchange between the server and client.
Queue and Job Processing Disruption
- Halts job dispatching.
- Prevents background task execution.
- May lead to the loss of critical application workflow tasks.
Data Serialization Blockage
- Prevents objects and arrays from being converted into JSON.
- Breaks data transfer mechanisms.
- Increases the risk of data loss during transmission.
Application Performance
- Causes unexpected system interruptions.
- Leads to an inconsistent user experience.
- Requires manual intervention to resolve the issue.
System Reliability
- Reduces overall application stability.
- Triggers unexpected exceptions.
- May expose potential system vulnerabilities.
Causes and Fixes
1. Non-Serializable Objects
Cause:
Occurs when passing objects with non-serializable properties or deeply nested structures.
Fix
- Implement the Serializable interface.
- Use the
__serialize()
and__unserialize()
methods. - Convert complex objects into simple arrays or scalar values before queueing.
Identify Non-Serializable Properties:
- Examine all object properties.
- Identify complex or non-primitive types.
- Determine which properties cannot be serialized.
Identify Non-Serializable Properties:
class UserData implements Serializable {
private $id;
private $name;
private $complexObject; // Potential serialization issue
public function serialize() {
return serialize([
'id' => $this->id,
'name' => $this->name,
'complexData' => $this->extractSerializableData()
]);
}
public function unserialize($data) {
$unserializedData = unserialize($data);
$this->id = $unserializedData['id'];
$this->name = $unserializedData['name'];
}
private function extractSerializableData() {
// Convert complex objects to simple, serializable format
return [
'key1' => $this->complexObject->getPrimitiveValue(),
'key2' => $this->complexObject->getSimpleRepresentation()
];
}
}
Key Considerations
- Utilize primitive data types whenever possible.
- Implement custom serialization logic for complex data structures.
- Carefully manage the conversion of complex objects so that proper serialization is ensured.
2. Binary/Non-Unicode Strings
Cause
Strings containing binary data or non-standard character encodings.
Fix
- Ensure proper character encoding is used.
- Use mb_convert_encoding() to normalize strings.
- Remove or replace problematic characters before processing.
$cleanString = mb_convert_encoding($originalString, 'UTF-8', 'auto');
Comprehensive Encoding Strategy
class StringHandler {
public static function sanitizeString($input) {
// Multiple encoding protection layers
$steps = [
'detect_encoding' => mb_detect_encoding($input, 'UTF-8, ISO-8859-1, Windows-1252', true),
'normalize' => mb_convert_encoding($input, 'UTF-8', 'auto'),
'remove_invalid' => preg_replace('/[^\x20-\x7E]/','', $input),
'trim_whitespace' => trim($input)
];
return $steps['normalize'];
}
}
Encoding Validation Techniques
- Use
mb_detect_encoding()
to identify character encoding. - Implement multi-step normalization for consistency.
- Remove potentially problematic characters before processing.
- Ensure all data is encoded in UTF-8 format.
3. Recursive References
Cause:
Objects containing circular references, leading to infinite recursion during JSON encoding.
Fix:
- Break circular references before serialization.
- Use the JsonSerializable interface for better control.
- Manually specify which properties should be serialized.
public function jsonSerialize() {
return [
'id' => $this->id,
'name' => $this->name
];
}
Advanced Circular Reference Prevention:
class SafeJsonSerializable implements JsonSerializable {
private $data;
private $visited = [];
public function jsonSerialize() {
return $this->serializeRecursive($this->data);
}
private function serializeRecursive($item) {
// Prevent infinite recursion
if (is_object($item)) {
$hash = spl_object_hash($item);
if (isset($this->visited[$hash])) {
return '[Circular Reference]';
}
$this->visited[$hash] = true;
}
if (is_array($item)) {
return array_map([$this, 'serializeRecursive'], $item);
}
return $item;
}
}
4. Resource Handles
Cause
Occurs when trying to serialize PHP resources, such as file handles or database connections.
Fix:
- Convert resources into storable identifiers (e.g., file paths, database IDs).
- Close resources before attempting serialization.
- Pass only the necessary data, rather than the entire resource object.
Example
Incorrect: Passing a file handle directly.
$fileHandle = fopen('example.txt', 'r'); // Not serializable
Correct: Pass the file path instead.
$filePath = $file->getPath();
Resource Management Strategy
class ResourceHandler {
private $filePath;
private $fileContents;
public function __construct($file) {
// Convert resource to storable data
if (is_resource($file)) {
$this->filePath = stream_get_meta_data($file)['uri'];
$this->fileContents = stream_get_contents($file);
fclose($file);
}
}
public function recreateResource() {
// Safely recreate resource if needed
return fopen($this->filePath, 'r');
}
}
5. Closures or Anonymous Functions
Cause
Fix:
- Avoid passing functions in queued jobs.
- Use static methods or named functions instead.
- Implement alternative design patterns to handle callbacks or deferred execution.
// Bad
$job = new ProcessJob(function() { /* complex logic */ });
// Good
$job = new ProcessJob($specificData);
Dependency Injection Alternative
class ProcessJob {
private $processData;
public function __construct(array $processData) {
$this->processData = $processData;
}
public function handle() {
// Use predefined data instead of dynamic closure
$this->executeProcess($this->processData);
}
private function executeProcess(array $data) {
// Process logic here
}
}
Prevention Strategies
Validate Payload Before Queueing
- Perform type checking to ensure data compatibility.
- Implement custom serialization methods for complex objects.
- Sanitize data before dispatching to prevent serialization errors.
Validate Payload Before Queueing
- Convert complex objects into simple scalar types before serialization.
- Implement the
__set_state()
method to handle complex object reconstruction.
Implement Error Handling
try {
// Queue job
} catch (InvalidPayloadException $e) {
// Log and handle gracefully
}
Regular Data Validation
- Create middleware to verify serialization before dispatching jobs.
- Use Laravel’s model observers for pre-processing and data preparation.
Monitoring and Logging
- Set up comprehensive logging to track serialization issues.
- Continuously monitor queue processing to identify problems.
- Create alerts to notify when serialization failures occur.
[Need assistance with a different issue? Our team is available 24/7.]
Conclusion
The “Unable to JSON encode payload. Error code: 5” in Laravel occurs when data cannot be serialized into JSON, affecting job dispatching and event handling. Addressing this involves fixing non-serializable objects, binary strings, and recursive references, along with implementing proper encoding and serialization methods. Prevention strategies like regular data validation and monitoring can help avoid these issues.
For quick resolution and expert assistance with Laravel-related errors, Bobcares Laravel support services provide reliable solutions, ensuring your application runs smoothly and efficiently, minimizing disruptions and optimizing performance.
0 Comments